This time I will share jQuery Plugin and tutorial about Create Flexible Dialog Boxes Using Bootstrap 4 – Bootprompt, hope it will help you in programming stack.
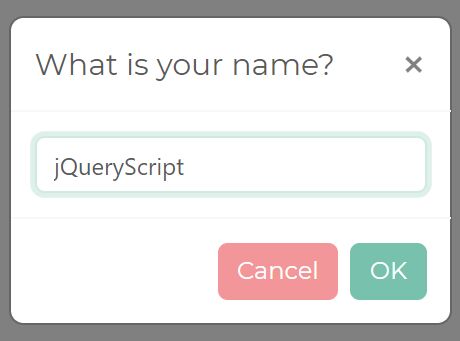
File Size: | 496 KB |
---|---|
Views Total: | 1647 |
Last Update: | |
Publish Date: | |
Official Website: | Go to website |
License: | MIT |
Bootprompt is the upgraded version of Bootbox plugin that lets you create flexible, multi-language alert, confirmation, prompt and custom popup boxes using the latest Bootstrap 4 or Bootstrap 3 framework.
How to use it:
1. Load the needed jQuery JavaScript library and Bootstrap framework in the document.
1 |
< link rel = "stylesheet" href = "/path/to/cdn/bootstrap.min.css" /> |
2 |
< script src = "/path/to/cdn/jquery.slim.min.js" ></ script > |
3 |
< script src = "/path/to/cdn/bootstrap.min.js" ></ script > |
2. Load the jQuery Bootprompt plugin from a CDN.
1 |
<!-- Core --> |
2 |
< script src = "/path/to/dist/bootprompt.min.js" ></ script > |
3 |
<!-- Locals--> |
4 |
< script src = "/path/to/dist/bootprompt.locales.min.js" ></ script > |
5 |
<!-- Or From A CDN --> |
6 |
< script src = "https://unpkg.com/bootprompt" ></ script > |
3. Create an alert popup box.
1 |
bootprompt.alert( "Alert!" ); |
2 |
// or |
3 |
bootprompt.alert({ |
4 |
size: "small" , |
5 |
title: "Dialog Title" , |
6 |
message: "Your message here…" , |
7 |
callback: function (){} |
8 |
}) |
4. Create a confirmation popup box.
01 |
bootprompt.confirm( "Are you sure?" , function (result){ |
02 |
alert( 'confirmed' ) |
03 |
}) |
04 |
// or |
05 |
bootprompt.confirm({ |
06 |
size: "small" , |
07 |
message: "Are you sure?" , |
08 |
callback: function (result){ |
09 |
alert( 'confirmed' ) |
10 |
} |
11 |
}) |
5. Create a prompt popup box.
01 |
bootprompt.prompt( "What is your name?" , function (result){ |
02 |
// do something |
03 |
}) |
04 |
// or |
05 |
bootprompt.prompt({ |
06 |
value: '' , // initial value |
07 |
inputType: 'input' , // any form elements |
08 |
inputOptions: {}, |
09 |
min: null , // min value |
10 |
max: null , // max value |
11 |
step: null , // step size |
12 |
maxlength: null , // max length |
13 |
pattern: '' , // require the input value to follow a specific format |
14 |
placeholder: '' , |
15 |
required: true , // if is required |
16 |
size: "small" , |
17 |
title: "What is your name?" , |
18 |
callback: function (result){ |
19 |
// result = String containing user input if OK clicked or null if Cancel clicked |
20 |
} |
21 |
}) |
6. Create a custom popup box.
1 |
bootprompt.dialog({ |
2 |
message: 'HTML content here' |
3 |
}) |
7. Global options with default values.
01 |
bootprompt.dialog({ |
02 |
03 |
// dialog message |
04 |
message: 'HTML content here' , |
05 |
06 |
// title |
07 |
title: 'dialog title' , |
08 |
09 |
// shows the dialog immediately |
10 |
show: true , |
11 |
12 |
// enables backdrop or not |
13 |
backdrop: null , |
14 |
15 |
// shows close button |
16 |
closeButton: true , |
17 |
18 |
// enables animations or not |
19 |
animate: true , |
20 |
21 |
// extra CSS class |
22 |
className: null , |
23 |
24 |
// dialog size |
25 |
size: 'small' , |
26 |
27 |
// flips the order in which the buttons are rendered, from cancel/confirm to confirm/cancel |
28 |
swapButtonOrder: false , |
29 |
30 |
// adds the the modal-dialog-centered to the doalog |
31 |
centerVertical: false , |
32 |
33 |
// dismisses the dialog by hitting ESC |
34 |
onEscape: true , |
35 |
36 |
// custom action buttons |
37 |
buttons: {}, |
38 |
39 |
// callback |
40 |
callback: function (){}, |
41 |
42 |
// allows the user to dismiss the dialog by pressing ESC |
43 |
onEscape: true |
44 |
|
45 |
}) |
8. The plugin also supports more than 38 locals.
- ar Arabic
- az Azerbaijani
- bg_BG Bulgarian
- br Portuguese – Brazil
- cs Czech
- da Danish
- de German
- el Greek
- en English
- es Spanish / Español
- et Estonian
- eu Basque
- fa Farsi / Persian
- fi Finnish
- fr French / Français
- he Hebrew
- hr Croatian
- hu Hungarian
- id Indonesian
- it Italian
- ja Japanese
- ko Korean
- lt Lithuanian
- lv Latvian
- nl Dutch
- no Norwegian
- pl Polish
- pt Portuguese
- ru Russian
- sk Slovak
- sl Slovenian
- sq Albanian
- sv Swedish
- th Thai
- tr Turkish
- uk Ukrainian
- zh_CN Chinese (People’s Republic of China)
- zh_TW Chinese (Taiwan / Republic of China)
1 |
bootprompt.dialog({ |
2 |
3 |
locale: 'en' |
4 |
5 |
}) |
9. API methods.